Model building – Vertex AI Workbench and Custom Model Training
Once the Dockerfile is created, we need to work on the Python code for the model building. The task is to build a regression model to predict the electricity prices. Python code will be copied into the container and submitted for training job.
Let us go back to the terminal of the workbench and follow these steps to create the python code for model building:
Step 1: Create trainer folder
Type the commands in the terminal as shown in Figure 4.16:
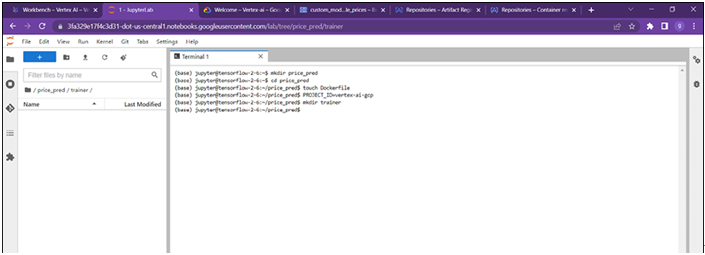
Figure 4.16: Trainer folder creation in terminal
PROJECT_ID=vertex-ai-gcp
mkdir trainer
In the first command, a variable called PROJECT_ID is set to the project ID (use project ID not the project name), we will use this command while creating a docker image. In the second line of command, we will create a new folder called trainer. Open the trainer folder.
Step 2: Create Python file
Follow the steps mentioned in Figure 4.17 to create Python file:
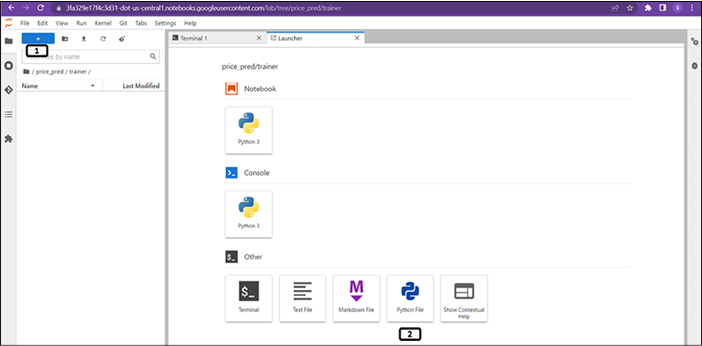
Figure 4.17: Python file creation on workbench
- Click New launcher.
- Double click on the Python File.
Step 3: Python code
Python file will be created inside the trainer folder, write the below given Python code in the py file as shown in the Figure 4.18:
import numpy as np
import pandas as pd
import pathlib
import tensorflow as tf
Importing tensorflow 2.6
from tensorflow import keras
from tensorflow.keras import layers
def main():
#Reading data from the gcs bucket
dataset=pd.read_csv(r”gs://custom_model_ele_prices/electricity_prices.csv”, low_memory=False)
dataset.tail()
BUCKET = ‘gs://custom_model_ele_prices’
dataset.isna().sum()
dataset = dataset.dropna()
dataset.drop([‘DateTime’, ‘Holiday’], axis=1,inplace=True)
cols = list(dataset.columns[dataset.dtypes.eq(‘object’)])
dataset[cols] = dataset[cols].apply(pd.to_numeric, errors=’coerce’, axis=1)
train_dataset = dataset.sample(frac=0.8,random_state=0)
test_dataset = dataset.drop(train_dataset.index)
train_stats = train_dataset.describe()
train_stats.pop(“SMPEP2”)
train_stats = train_stats.transpose()
train_labels = train_dataset.pop(‘SMPEP2’)
test_labels = test_dataset.pop(‘SMPEP2’)
normed_train_data =(train_dataset-train_dataset.mean())/train_dataset.std()
normed_test_data=(test_dataset-train_dataset.mean())/train_dataset.std()
def tf_build_model_reg():
#model building function
model = keras.Sequential([
layers.Dense(64, activation=’relu’, input_shape=[len(train_dataset.keys())]),
layers.Dense(64, activation=’relu’),
layers.Dense(128, activation=’relu’),
layers.Dense(32, activation=’relu’),
layers.Dense(1)])
optimizer = tf.keras.optimizers.Adagrad(0.001)
model.compile(loss=’mse’,optimizer=optimizer, metrics=[‘mae’, ‘mse’])
return model
model = tf_build_model_reg()
epochs = 10
early_stop = keras.callbacks.EarlyStopping(monitor=’val_loss’, patience=5)
early_history = model.fit(normed_train_data, train_labels,epochs=epochs, validation_split = 0.2,callbacks=[early_stop])
model.save(BUCKET + ‘/model’)
if name==”main”:
main()
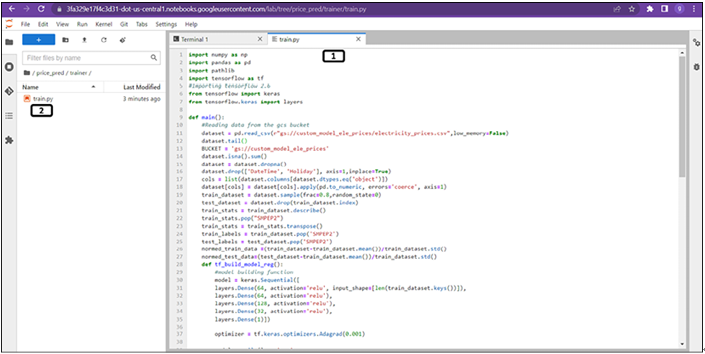
Figure 4.18: Python code for model training
- Type the Python code.
- Right click and rename the Python file name to train.py.
Save the Python file (Ctrl + S) and close the file.
Python code consists of code to import required packages. Main function reads the .csv file from the cloud storage bucket apply few data transformations, split the data for train and test. tf_build_model_reg function contains the skeleton for the TensorFlow model (model will be built using TensorFlow 2.6). At the last, trained model will be pushed into the cloud storage bucket.
Note: We have used Epochs to be 10. Increase it as per your need.
The code used for this exercise is not very detailed since it is only for illustrative purposes. Users can use more complex code for the model training.
You may also like
Archives
- September 2024
- August 2024
- July 2024
- June 2024
- May 2024
- April 2024
- March 2024
- February 2024
- January 2024
- December 2023
- November 2023
- September 2023
- August 2023
- June 2023
- May 2023
- April 2023
- February 2023
- January 2023
- November 2022
- October 2022
- September 2022
- August 2022
- June 2022
- April 2022
- March 2022
- February 2022
- January 2022
- December 2021
- November 2021
- October 2021
Calendar
M | T | W | T | F | S | S |
---|---|---|---|---|---|---|
1 | 2 | |||||
3 | 4 | 5 | 6 | 7 | 8 | 9 |
10 | 11 | 12 | 13 | 14 | 15 | 16 |
17 | 18 | 19 | 20 | 21 | 22 | 23 |
24 | 25 | 26 | 27 | 28 |
Leave a Reply