Submitting training job with Python SDK – Vertex AI Custom Model Hyperparameter and Deployment
In all the exercises that we have covered in the previous chapters, we have mainly worked on graphical user interface of the platform. Let us now see how to submit the job for training using Python code.
Step 1: Create a new python notebook on the Jupyter lab
Follow the given steps to create a new Python notebook file, as shown in the following figure:

Figure 5.32: Creation of Python notebook
Follow the corresponding points:
- Click on new launcher, that is, the + sign.
- Click on Python notebook to create one.
Step 2: Python code for the training job initiation
Cell 1:
!pip3 install google-cloud-aiplatform –upgrade –user
from google.cloud import aiplatform
from google.cloud.aiplatform import hyperparameter_tuning as hpt
Cell 2:
worker_pool_details = [{
“machine_spec”: {
“machine_type”: “n1-standard-4”
},
“replica_count”: 1,
“container_spec”: {
“image_url”: “gcr.io/vertex-ai-gcp-1/eye:v1”
}
}]
metric_spec={‘accuracy’:’maximize’} #Metric to be optimized
Hyperparameters which needs to be optimized
parameter_spec_list = {“n_estimators”: hpt.DiscreteParameterSpec(values=[100,125], scale=None),
“max_depth”: hpt.DiscreteParameterSpec(values=[5,10,15,20], scale=None),
“min_samples_split”: hpt.DiscreteParameterSpec(values=[5,10], scale=None),
“min_samples_leaf”:hpt.DiscreteParameterSpec(values=[5,10], scale=None),
“max_features”:hpt.CategoricalParameterSpec(values=[“auto”,”sqrt”])}
Cell 3:
my_custom_job = aiplatform.CustomJob(display_name=’eye_classification-sdk-job’,worker_pool_specs=worker_pool_details,staging_bucket=’gs://hpo_vertex-ai’)
Cell 4:
job_hyperparameter = aiplatform.HyperparameterTuningJob(
display_name=’eye_classification-sdk-job’,
custom_job=my_custom_job,
metric_spec=metric_spec,
parameter_spec=parameter_spec_list,
max_trial_count=2,
parallel_trial_count=1)
job_hyperparameter.run() #To initiate the hyperparameyter tuning job
Enter the code given previously, in different cells, as shown in the Figure 5.33:
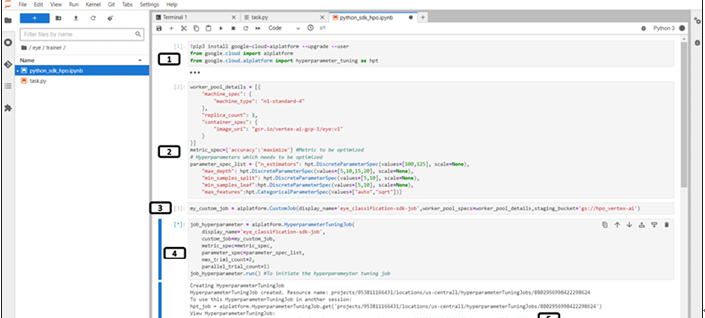
Figure 5.33: Python code for submitting training job
Refer to the corresponding points for a better understanding of what each code does in each cell:
- Installing and importing the required packages. Even though we are using notebook on GCP, google-cloud-ai platform will not be pre-installed.
- Defining the hardware configuration, metrics to optimize and hyperparameter list.
- Defining a custom job with display name and other details (if custom job needs to be initiated without hyperparameter optimization then add my_custom_job.run() to initiate the custom model training).
- Adding hyperparameter step to the custom job training defined in the previous step.
- Click on the link. It will take to the training page, as shown in Figure 5.34:
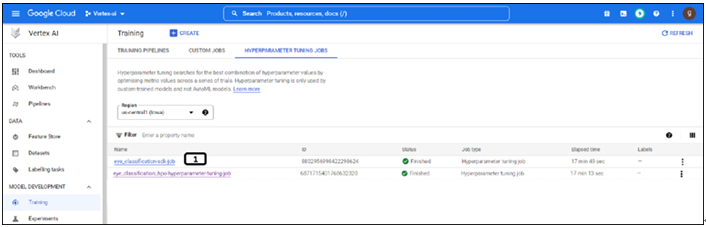
Figure 5.34: Training successfully completed
Deletion of resources
All the compute resources are expensive. Follow the steps mentioned in Chapter 2 under Model un-deployment and deleting end point to un-deploy model and endpoint deletions. Follow the steps mentioned in Chapter 2 under Model deletion to delete model from registry. Follow the steps mentioned in Chapter 4 under Deletion of resources to delete the workbench instance.
Conclusion
In this chapter, we learned about the custom model in detail, along with the hyper parameter tuning. We also covered how to submit the custom model for training using both GUI and SDK methods, and how to import the train models to model registry.
In the next chapter, we will start working on the pipelines of GCP.
Questions
- How to pass the parameters for tuning in custom job?
- During the initiation of training job including hyperparameter tuning from python SDK, why is defining custom training job needed?
- If we are training model using PyTorch package, can we use pre-built containers for training?
You may also like
Archives
- September 2024
- August 2024
- July 2024
- June 2024
- May 2024
- April 2024
- March 2024
- February 2024
- January 2024
- December 2023
- November 2023
- September 2023
- August 2023
- June 2023
- May 2023
- April 2023
- February 2023
- January 2023
- November 2022
- October 2022
- September 2022
- August 2022
- June 2022
- April 2022
- March 2022
- February 2022
- January 2022
- December 2021
- November 2021
- October 2021
Calendar
M | T | W | T | F | S | S |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 | 31 |
Leave a Reply